What is Express.js?
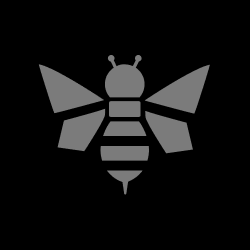
Hire Arrive
Technology
5 months ago
Express.js, often shortened to just Express, is a minimalist and flexible Node.js web application framework. It's renowned for its speed, efficiency, and ease of use, making it a popular choice for building everything from simple APIs to complex web applications and mobile backends. Think of it as a powerful toolbox that streamlines the process of creating server-side logic for your applications.
While Node.js provides the foundation for running JavaScript on the server, Express.js builds upon it, offering a structured and organized way to handle requests and responses. It doesn't dictate a specific architecture or methodology, giving developers significant freedom in designing their applications. This flexibility is a key part of its appeal.
Key Features and Benefits:
* Minimalist and Unopinionated: Express.js avoids imposing strict conventions or architectural patterns. This allows developers to choose the tools and techniques best suited to their project. * Fast and Efficient: Built on Node.js's non-blocking, event-driven architecture, Express.js handles many concurrent requests efficiently, making it suitable for high-traffic applications. * Routing: Express.js provides a robust routing mechanism allowing you to define how the server responds to different HTTP requests (GET, POST, PUT, DELETE, etc.) based on the URL and HTTP method. * Middleware: A powerful feature allowing you to add functionalities to your application in a modular and reusable way. Middleware functions execute before reaching the request handler, enabling tasks like authentication, logging, and data parsing. * Templating Engines: Express.js integrates seamlessly with various templating engines (like Pug, EJS, Handlebars) for generating dynamic HTML content. * Large and Active Community: A large and active community provides extensive support, documentation, and readily available third-party packages. * Easy to Learn: Its relatively simple structure and clear documentation make it accessible to both beginners and experienced developers.
How Express.js Works:
At its core, Express.js sits between the client (e.g., a web browser) and the Node.js server. When a client makes a request, Express.js intercepts it, processes it through its middleware stack, and then routes it to the appropriate handler function. This handler function performs the necessary actions (e.g., database queries, data manipulation) and sends a response back to the client.
Example (Simple Hello World):
```javascript const express = require('express'); const app = express(); const port = 3000;
app.get('/', (req, res) => { res.send('Hello from Express.js!'); });
app.listen(port, () => { console.log(`Server listening on port ${port}`); }); ```
This simple code snippet shows how to create a basic Express.js server that responds with "Hello from Express.js!" when a GET request is made to the root URL.
When to Use Express.js:
Express.js is a versatile framework suitable for a wide range of projects, including:
* RESTful APIs: Building robust and scalable APIs for mobile and web applications. * Single-Page Applications (SPAs): Serving data and handling requests for SPAs built with frameworks like React, Angular, or Vue.js. * Web Applications: Developing complete web applications, though often paired with other tools for front-end rendering. * Microservices: Constructing individual, independent services within a larger application architecture.
In conclusion, Express.js is a powerful and flexible framework that simplifies the process of building server-side applications with Node.js. Its minimalist nature, ease of use, and extensive community support make it a compelling choice for developers of all skill levels.